Extending Redis with Redis-Modules
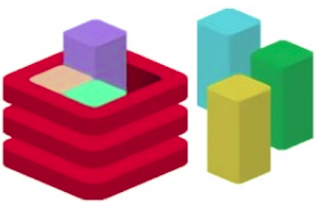
Redis is a great K/V store written in C. Redis can accomplish a lot for a single thread process. There are many applications for redis like for instance:
- Frontend Database- Real-time Counters
- Ad Serving
- Message Queue
- Geo and TimeSeries DB
- Session State
- Cache
That's all great but let's say I want more, how can we customize redis? There are some options like a Lua Script, Fork Redis, Tak to @Antirez or Create your own NoSQL database based on redis or not. Redis provided another solution - Redis added support for external modules in 2016.
Redis Modules
Redis Modules are just dynamic libraries(.so files) loaded on redis - Often these libraries are written in C or C++ but there are some bindings where you write modules in Go or JavaScript for instance.
The best news is if you write one library you have ZERO LATENCY to access data in Redis - So this is a very big win and strong reason to write your own library.
Redis Modules enable a whole new set of extensions for you. For instance is possible to create new Datatypes. Its possible to create new Commands. It's possible also to combine existing commands and add custom new functionality using an existent command, datatypes in order to archive your goals.
There is just 1 bad news. Remember is C. C often can be fragile so you need to have great discipline and test very well your code. One wrong thing can tear down the whole redis process and I don't need to say how bad this is.
Redis Modules are supported by version 4.0. There are some interesting available models already like this ones here:
- RediSearch - Search support for Redis
- ReJSON - JSON data type and operations support for Redis
- Redis-ML - Machine Learning with Redis
- rebloom - Scalable Bloom Filters for Redis.
There are some dependencies in order to build and run the redis module. Make sure you have installed:
- docker
- redis-cli
- build-essential
- RediSearch - Search support for Redis
- ReJSON - JSON data type and operations support for Redis
- Redis-ML - Machine Learning with Redis
- rebloom - Scalable Bloom Filters for Redis.
- docker
- redis-cli
- build-essential
So let's start with the Dockerfile. We will use docker to run redis 4.0 with the custom module we will build. Let's define the Dockerfile.
Dockerfile
date.c
RedisModule_OnLoad: We use this function to load the module and all commands.
diegoDate_RedisCommand: The command implementation code.
getDate: A helper function which returns the current date as string.
As you might realize there is an include for redismodule.h which is the redis module API we need to interact with Redis.
redismodule.h
Makefile
Running
Now in bash, we can do:
$ make clean
$ make
$ make docker
$ make run
$ make clean
$ make
$ make docker
$ make run
This will build the module and bake the docker image and run the docker container. After doing that we can open redis-cli by doing: $ redis-cli
So finally we can test our command by doing $ dp.DATE and you should see the current date. That's it, folks, we have a very simple redis module working.
If you want have the full code you can get on my github here.
Cheers,
Diego Pacheco